1、设计思路:
主要采用SharedPreferences来保存用户数据,本Demo没有经过加密,所有一旦Android系统被ROOT的话,其他用户就可以查看用户的私有目录,密码文件就很不安全。所以真正应用在软件上面的,一定要经过加密才保存,可以选择MD5加密。
SharedPreferences介绍可以参看这篇博文:http://blog.csdn.net/conowen/article/details/7312612
TextWatcher的介绍可以参看这篇博文:http://blog.csdn.net/conowen/article/details/7420673
2、功能介绍
默认勾选“记住密码”复选框,点击“登陆”按钮,一旦成功登陆,就保存用户名和密码到SharedPreferences文件中。
用户名输入时,通过TextWatcher不断去读取用户数据,自动提示相应的“用户名”,选择了用户名之后,就会读取SharedPreferences的文件,然后自动完成密码的输入。
3、效果图:
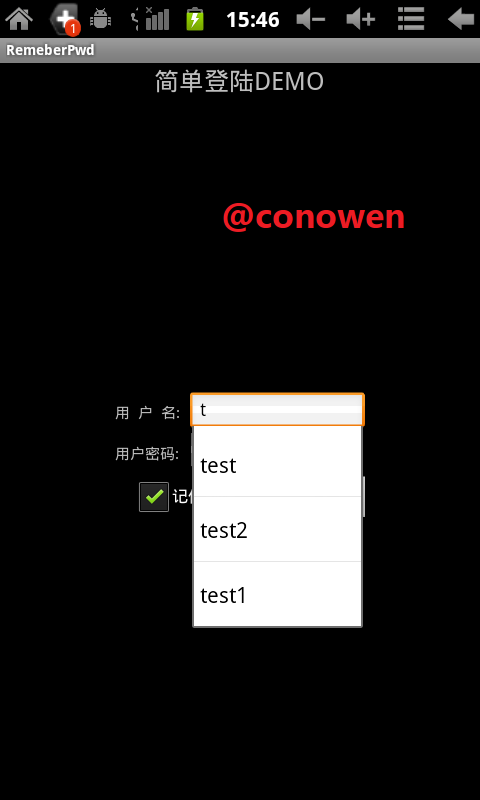

4、代码:详细都在注释里面了
-
-
-
-
-
packagecom.conowen.remeberPwd;
-
-
importandroid.app.Activity;
-
importandroid.content.SharedPreferences;
-
importandroid.os.Bundle;
-
importandroid.text.Editable;
-
importandroid.text.InputType;
-
importandroid.text.TextWatcher;
-
importandroid.view.View;
-
importandroid.view.View.OnClickListener;
-
importandroid.widget.ArrayAdapter;
-
importandroid.widget.AutoCompleteTextView;
-
importandroid.widget.Button;
-
importandroid.widget.CheckBox;
-
importandroid.widget.EditText;
-
importandroid.widget.Toast;
-
-
publicclassRemeberPwdActivityextendsActivity{
-
-
AutoCompleteTextViewcardNumAuto;
-
EditTextpasswordET;
-
ButtonlogBT;
-
-
CheckBoxsavePasswordCB;
-
SharedPreferencessp;
-
StringcardNumStr;
-
StringpasswordStr;
-
-
-
@Override
-
publicvoidonCreate(BundlesavedInstanceState){
-
super.onCreate(savedInstanceState);
-
setContentView(R.layout.main);
-
cardNumAuto=(AutoCompleteTextView)findViewById(R.id.cardNumAuto);
-
passwordET=(EditText)findViewById(R.id.passwordET);
-
logBT=(Button)findViewById(R.id.logBT);
-
-
sp=this.getSharedPreferences("passwordFile",MODE_PRIVATE);
-
savePasswordCB=(CheckBox)findViewById(R.id.savePasswordCB);
-
savePasswordCB.setChecked(true);
-
cardNumAuto.setThreshold(1);
-
passwordET.setInputType(InputType.TYPE_CLASS_TEXT
-
|InputType.TYPE_TEXT_VARIATION_PASSWORD);
-
-
-
-
cardNumAuto.addTextChangedListener(newTextWatcher(){
-
-
@Override
-
publicvoidonTextChanged(CharSequences,intstart,intbefore,
-
intcount){
-
-
String[]allUserName=newString[sp.getAll().size()];
-
allUserName=sp.getAll().keySet().toArray(newString[0]);
-
-
-
-
-
ArrayAdapter<String>adapter=newArrayAdapter<String>(
-
RemeberPwdActivity.this,
-
android.R.layout.simple_dropdown_item_1line,
-
allUserName);
-
-
cardNumAuto.setAdapter(adapter);
-
-
}
-
-
@Override
-
publicvoidbeforeTextChanged(CharSequences,intstart,intcount,
-
intafter){
-
-
-
}
-
-
@Override
-
publicvoidafterTextChanged(Editables){
-
-
passwordET.setText(sp.getString(cardNumAuto.getText()
-
.toString(),""));
-
-
}
-
});
-
-
-
logBT.setOnClickListener(newOnClickListener(){
-
-
@Override
-
publicvoidonClick(Viewv){
-
-
-
cardNumStr=cardNumAuto.getText().toString();
-
passwordStr=passwordET.getText().toString();
-
-
if(!((cardNumStr.equals("test"))&&(passwordStr
-
.equals("test")))){
-
Toast.makeText(RemeberPwdActivity.this,"密码错误,请重新输入",
-
Toast.LENGTH_SHORT).show();
-
}else{
-
if(savePasswordCB.isChecked()){
-
sp.edit().putString(cardNumStr,passwordStr).commit();
-
}
-
Toast.makeText(RemeberPwdActivity.this,"登陆成功,正在获取用户数据……",
-
Toast.LENGTH_SHORT).show();
-
-
-
-
}
-
-
}
-
});
-
-
}
-
-
}
布局文件:main.xml
SharedPreferences文件,在/data/data/包名/shared_prefs文件夹下面
-
<?xmlversion='1.0'encoding='utf-8'standalone='yes'?>
-
<map>
-
<stringname="test">test</string>
-
<stringname="test2">test</string>
-
<stringname="test1">test</string>
-
</map>
分享到:
相关推荐
这段源码直接使用SharedPreferences类。就可以达到保存用户名和密码的功能,代码很短,很容易读懂。移植性很好。
android studio 第五章课后实践(实现登录界面设计、记住密码操作) 适用 学生
主要为大家详细介绍了Android实现带有记住密码功能的登陆界面,主要采用SharedPreferences来保存用户数据,感兴趣的小伙伴们可以参考一下
Android记住密码和自动登录实现,本人已经亲测成功,Android2.3.3运行环境。
android登陆界面设计,记住密码功能,登陆密码成功后进入APP
Android登陆界面记住账号密码,有更好求分享
android 模仿qq登录界面EditText下拉框记住账号和密码 editText+popupwindow+sqlite方式实现
这个程序是一个Android的登陆界面的设计,它实现了如下功能:记住密码,用户输入时有用户名提示选择。还有管理员界面,添加新用户,修改密码。这里使用的是sharedpreferences和数据库两种方式。
简单可用的登录示例,为了避免以后忘记,上传至此。
非常好的登陆界面,android开发,记住密码
android记住密码自动登录.zip tablelogin(登陆界面).rar 一个登陆和注册界面.rar 一个简单注册界面.rar 一个简单登录的DEMO.rar 仿QQ微信登录页面.rar 用户注册,登录的简单实现.zip 自动清空edittext.rar 通过...
SharedPreference方面的内容还算是比较简单易懂的,在此还是主要贴上效果与代码,最后也是附上源码。
登陆界面创建一个复选按钮,通过按钮选取来进行事件处理。若按钮选中记住账号和密码的信息,本文教大家如何使用Android实现记住用户名和密码功能,感兴趣的小伙伴们可以参考一下
本小程序实现了一个登陆界面的布置,当用户启动程序时输入用户名和密码,点击记住密码当下次在次启动程序时密码将自动的保留,不用输入。界面比较简单,希望大家可以继续的完善一下。希望能给大家带来帮助。 效果图...
这个资源实现了login和register登陆界面设计 并且采用SharedPreferences+Broadcast Receiver+Service实现了以下功能: 1.用户登录 2.用户注册 3.用户记住密码 4.用户自动登录 这个资源花了我很长时间 里卖用到了...
数据持久化就是指将那些内存中的瞬时数据保存到持久化设备中(如手机文件、数据库等),当关机,停电后,数据不丢失。 Android 系统中主要提供了三...这个文件是使用SharedPreference 存储 实现登陆时记住密码的小demo
Android源码方便学习,代码是最好的老师。
欢迎界面登陆后有提示音,实现本地音乐播放暂停,上一首下一首,单曲循环,随机播放,顺序播放,歌词同步,摇一摇切歌功能,音量控制,有歌曲艺术家分类,播放界面,集于一体的强大音乐播放器很适合初学者。
登录可以选择记住密码和自动登陆两种选择。 点击底部的+可以添加便笺,默认点击返回键会自动保存。 点击便笺列表可以进入便笺编辑界面,对便笺进行编辑,默认点击返回键会自动保存。 长按便笺列表的某一项,可以唤出...